Archive for May, 2023
C – BASS lib example on how to play XM files
by admin on May.07, 2023, under News
The un4seen BASS library is a powerful, versatile, and efficient audio library designed to provide developers with a wide range of functionalities for handling and manipulating audio streams, samples, and music files. It is available for multiple platforms, including Windows, macOS, Linux, iOS, and Android, and supports various programming languages such as C/C++, C#, Delphi, and more. BASS offers a simple and intuitive API that allows developers to quickly integrate audio playback, recording, and processing capabilities into their applications. It supports a wide range of audio formats, including MP3, OGG, WAV, AIFF, and tracker formats such as MOD, S3M, XM, and IT. Additionally, the library’s functionality can be extended through plugins for formats like FLAC, WMA, MIDI, and others. Today we’ll focus on playing an XM file using this library.
First we download the latest version of the library from the website and extract it to a folder of out liking. For macOS we’d get a folder called bass24-osx as of today. We then create a C application that uses the library:
#include <stdio.h> #include <stdlib.h> #include <bass.h> void checkError() { DWORD error = BASS_ErrorGetCode(); if (error != BASS_OK) { printf("Error code: %d\n", error); exit(EXIT_FAILURE); } } int main(int argc, char *argv[]) { if (argc < 2) { printf("Usage: %s <path_to_xm_file>\n", argv[0]); return EXIT_FAILURE; } if (!BASS_Init(-1, 44100, 0, 0, NULL)) { checkError(); } HSTREAM xmStream = BASS_MusicLoad(FALSE, argv[1], 0, 0, BASS_MUSIC_RAMPS | BASS_SAMPLE_LOOP | BASS_SAMPLE_FLOAT, 1); checkError(); if (!BASS_ChannelPlay(xmStream, FALSE)) { checkError(); } printf("Playing XM file. Press any key to exit...\n"); getchar(); BASS_StreamFree(xmStream); BASS_Free(); return EXIT_SUCCESS; }
We may now use a C compiler such as clang or gcc to compile the program:
clang xm_player.c -o xm_player \ -lbass -I bass24-osx/ -L bass24-osx/ -Wl,-rpath,'.'
A short explanation of the used compiler flags:
- -o specifies the name of the output binary
- -lbass tells the compiler we’re using the library libbass.dylib
- -I tells the compiler where to find the include files (header files)
- -L tells the compiler where to find the library files (in this case libbass.dylib).
@rpath
is a dynamic linker feature that allows specifying relative library search paths in a compiled binary. It is used in conjunction with the-Wl,-rpath
linker flag during compilation. When a binary with@rpath
is executed, the dynamic linker searches for required libraries in the specified paths relative to the binary’s location. This enables greater flexibility in distributing applications with bundled libraries, avoiding the need for absolute library paths or modifying system library search paths.@rpath
is particularly useful for creating portable applications and simplifies library management for users, as they can run applications without worrying about library installation or configuration.
Overall, our setup looks like this:
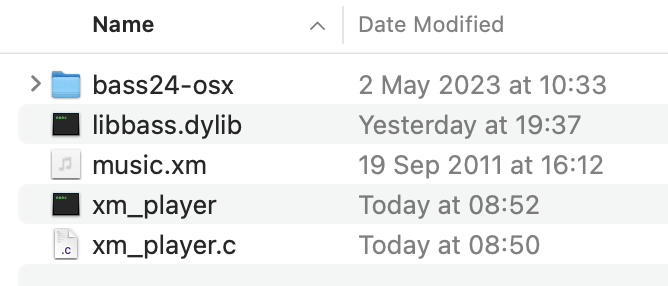
We can now play music by calling the program using the command ./xm_player music.xm